Comments in Python play a crucial role in programming by making code more readable, maintainable, and easier to debug. In Python, comments are non-executable lines that help developers understand the purpose of different parts of the code. They are especially useful when working in teams or revisiting code after a long time.
In this blog, we’ll explore the different types of comments in Python, best practices for writing effective comments, and how they impact performance.
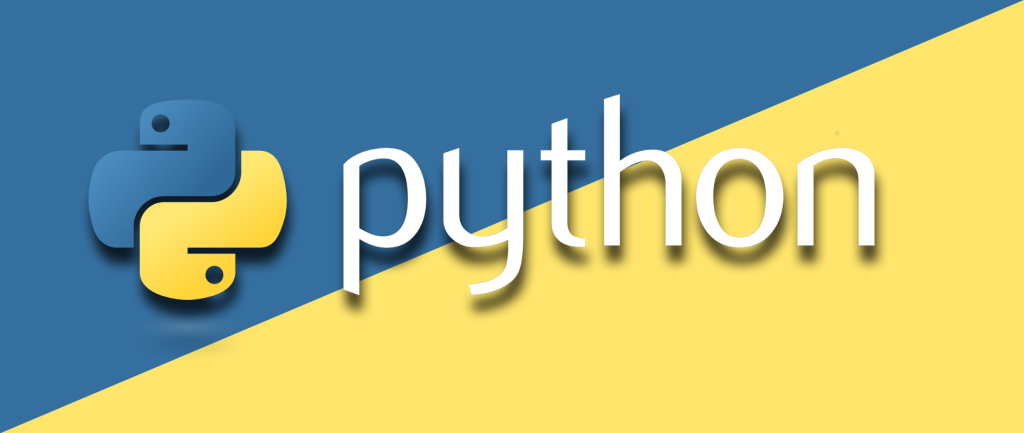
Table of Contents
What Are Comments in Python?
Comments in Python is a piece of text that is ignored by the interpreter. It does not affect the execution of the program but helps document the code.
For example:
# This is a comment
print("Hello, World!") # This prints a message
Here, the text following #
is a comment, and Python ignores it while executing the program.
Types of Comments in Python
Python supports three types of comments:
- Single-Line Comments
- Multi-Line Comments (Docstrings)
- Inline Comments
Let’s explore each of them in detail.
1. Single-Line Comments
A single-line comment starts with a #
symbol and continues until the end of the line.
Example:
# This is a single-line comment
x = 10 # Assigning 10 to x
Use single-line comments to:
- Explain a specific line of code
- Temporarily disable a line of code
# print("This line won't execute")
print("This will execute")
2. Multi-Line Comments (Using Triple Quotes or Multiple #
)
Python does not have a specific syntax for multi-line comments like other languages (e.g., /* ... */
in C or Java). However, we can achieve multi-line comments in two ways:
A) Using Multiple #
Symbols
# This is a multi-line comment
# explaining that the following code
# calculates the area of a rectangle.
length = 5
width = 3
area = length * width
print(area)
B) Using Triple Quotes ('''
or """
)
Triple quotes ('''
or """
) are primarily used for docstrings but can also serve as multi-line comments.
"""
This is a multi-line comment.
It describes the function below.
"""
def add(a, b):
return a + b
Note:
Using triple quotes this way is a workaround. Python treats them as strings, but if they are not assigned to a variable or used as docstrings, they are ignored.
3. Inline Comments
An inline comment appears on the same line as the code, separated by at least two spaces for better readability.
Example:
x = 100 # This variable stores the balance amount
y = x * 2 # Doubling the value of x
Use inline comments sparingly to explain complex logic, but avoid overusing them for obvious statements.
Bad Example:
x = 10 # Assign 10 to x
y = 20 # Assign 20 to y
(This is unnecessary since the code is already self-explanatory.)
Good Example:
x = 10 # Initializing x with a default value
Docstrings: Comments for Functions, Classes, and Modules
Python provides a special type of comment called docstrings (documentation strings) used to describe functions, classes, and modules. These are enclosed in triple quotes ("""
or '''
) and can span multiple lines.
1. Function Docstrings
def greet(name):
"""This function takes a name as input and returns a greeting message."""
return f"Hello, {name}!"
You can access the docstring using the __doc__
attribute:
print(greet.__doc__)
2. Class Docstrings
Animal:
"""This class represents an Animal with a name and sound."""
def __init__(self, name, sound):
self.name = name
self.sound = sound
3. Module-Level Docstrings
At the beginning of a Python file, you can include a docstring to describe the module.
"""
This module contains utility functions for mathematical operations.
Author: John Doe
Date: 2025-02-19
"""
Learn: How to Get Jobs in AI: A Comprehensive Guide
Best Practices for Writing Comments in Python
- Write meaningful comments:
- Instead of:
# Increment x by 1 x = x + 1
- Write:
# Updating the count of processed items x = x + 1
- Instead of:
- Use docstrings for functions and classes:
- Instead of:
# Function to add two numbers def add(a, b): return a + b
- Write:
def add(a, b): """Returns the sum of two numbers.""" return a + b
- Instead of:
- Keep comments concise and clear:
- Avoid unnecessary long comments that describe obvious things.
- Example:
# The following function calculates the factorial of a number using recursion def factorial(n): """Calculates the factorial of a number using recursion.""" return 1 if n == 0 else n * factorial(n - 1)
- Use comments for complex logic and business rules:
# If user is an admin, grant access to all sections if user_role == "admin": access_level = "full"
- Avoid redundant comments:
# Loop through the list for item in items: print(item)
Do Comments Affect Performance?
No!
Python ignores comments at runtime, so they do not affect performance. They are purely for documentation purposes.
However, excessive or unnecessary comments can reduce code readability. Strive for clean, self-explanatory code with minimal but effective comments.
When to Use Comments?
- Use comments when:
- Explaining complex logic
- Documenting functions, classes, or modules
- Clarifying why a specific approach is used
- Leaving reminders for future improvements
- Avoid comments when:
- The code is self-explanatory
- Writing redundant information
Conclusion
Comments in Python are powerful tools that improve code clarity, maintainability, and collaboration. By following best practices and using comments effectively, you can make your Python code more readable and professional.
- Use single-line comments for quick explanations.
- Use multi-line comments for longer descriptions.
- Use docstrings to document functions, classes, and modules.
- Keep comments meaningful, concise, and relevant.
Start writing clean and well-documented code today!
Learn:
Data Types in Python
Understanding Variables in Python: A Comprehensive Guide